REST APIs - HTTP is for More Than Web Browsing
Local Workstation Setup
• Linux utility curl
is used to send basic REST API requests
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
What is REST?
Overview
• REST stands for REpresentational State Transfer
• REST is an API framework built on HTTP
• REST is Just another user for the HTTP protocol besides web browsing
• API often referred to as Web Services
• Popular due to performance, scale and reliability
Requsts and Response, the REST API Flow
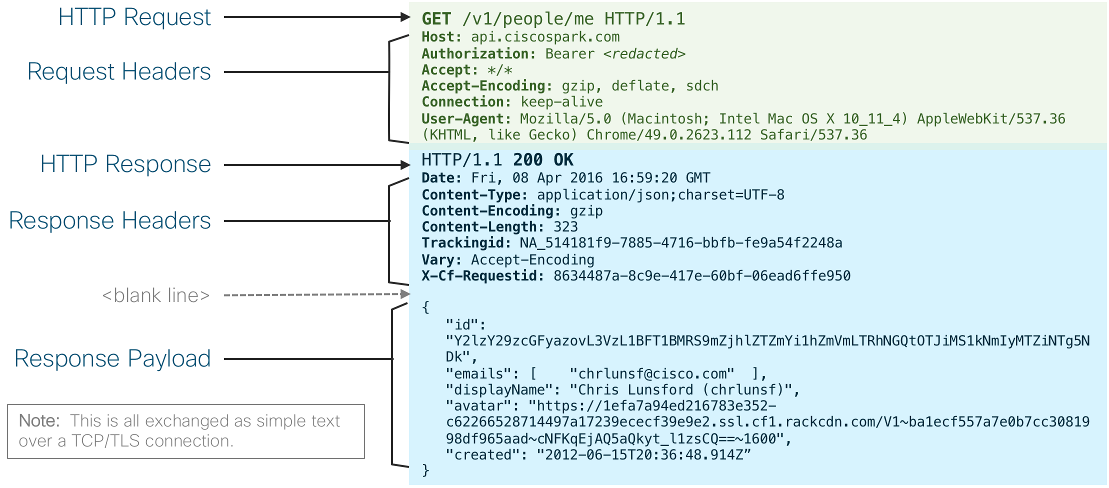
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
A Look Under the Hood at REST
The URI: What are you Requesting?
• An endpoint is the URI where you send an HTTP request.
<!-- Example: Endpoint (Google Maps API) -->
http://maps.googleapi.com/maps/api/geocode/json?address="sanjose"
| | | |
< Server or Host ><----- Resource -----><-- Parameters--->
Components of an URI:
URI Component | Description |
---|
Protocol | Define whether the host is open (http) or secured (https) |
Domain Name of Server/Host | Resolves to the IP to connect to, and may include port number |
Resource Path | The location of the data or object of interest on the server |
Parameters | Details to scope, filter, or clarify a request. Often optional |
HTTP Methods: What to Ask ?
• REST design centers around the HTTP request and response model. Consuming an API is as simple as making an HTTP request

• To start, determine which HTTP verb to use
HTTP Verb | Typical Purpose (CRUD) |
---|
POST | Create |
GET | Read |
PUT | Update |
PATCH | Update (tiny) |
DELETE | Delete |
Response Status Codes: Did the Request Work?
• After you or your application send a request that contains the correct URI and HTTP verb, the service returns a status code
Status Code | Status Message | Meaning |
---|
200 | OK | All looks good |
404 | Not found | Resources not found |
201 | Created | New resource created |
400 | Bad request | Request was invalid |
401 | Unauthorized | Authorization missing or incorrect |
403 | Forbidden | Request was understood but not allowed |
500 | Internal Server Error | Something wrong with the server |
503 | Service unavailable | Sever is unable to complete request |
REST API Headers: Details and Meta-data
Header | Example Value | Purpose |
---|
Content-Type | application/json | Specify the format of the data in the body (json/XML) |
Accept | application/json | Specify the requested format for reutrned data |
Authorization | Basic dmFncrosiIe?YW5 | Provide credentials to authorize a request |
Date | Tue, 25 Jul 2017 19:26:00 GMT | Date and time of the message |
• Used to pass information between client and server
• Included in both REQUEST and RESPONSE
• Some APIs will use custom headers for authentication or other purpose
REST API Authentication and Security
Who can use this REST API?
List of authentication methods:
Authentication Methods | Descriptions | Authorization Header |
---|
None | The Web API resource is public, anybody can place call | N/A |
Basic HTTP | A username and password are passed to the server in an encoded string | Basic ENCODESTRING |
Token | A secret generally retrieved from the Web API developer portal. Keyword (i.e. token) is API dependent | Token asdfjasldfjaslkd8as |
OAuth | Standard framework for a flow to retrieve an access token from an Identity Provider | Bearer us8asdkf91sdfj |
• Authorization can be short-lived and require freshing of tokens
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
REST Payloads
Data: Sending and Receiving
• Contained in the body
• POST, PUT, PATCH requests typically include data
• GET responses will include data
• The data format of the payload is typically JSON or XML
• You can check “Content-Type” header to know for certain what the service is sending. Look for Content-Type: application/json
, for example, indicating the service expects to see JSON
# Example: JSON object
{
'title': 'Hamlet',
'author': 'Shakespeare'
}
<!-- Example: XML from YANG model -->
<interfaces xmlns="urn:ietf:params:xml:ns:yang:ietf-interfaces">
<interface>
<name>GigabitEthernet1</name>
<type xmlns:ianaift="urn:ietf:params:xml:ns:yang:iana-if-type">ianaift:ethernetCsmacd</type>
<enabled>true</enabled>
<ipv4 xmlns="urn:ietf:params:xml:ns:yang:ietf-ip">
<address>
<ip>198.18.133.212</ip>
<netmask>255.255.192.0</netmask>
</address>
</ipv4>
<ipv6 xmlns="urn:ietf:params:xml:ns:yang:ietf-ip"/>
</interface>
...
</interfaces>
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Some REST API Examples
Syntax
• You can use the curl
tool to run these examples on Linux
# Syntax
curl https://<host>/<resource>?[parameter]
Public, no authentication required
• Example: The Internet Chuck Norris Database
# Example: Random Chuck Norris Joke
curl https://api.icndb.com/jokes/random
# Response
{ "type": "success", "value": { "id": 265, "joke": "The phrase 'balls to the wall' was originally conceived to describe Chuck Norris entering any building smaller than an aircraft hangar.", "categories": [] }
• When you have Python installed in your terminal, you can get nicer formatted JSON by piping the output to the Python JSON tool like so:
# Example: Get nicer output by piping
curl https://api.icndb.com/jokes/random | python -m json.tool
# Response
{
"type": "success",
"value": {
"categories": [],
"id": 442,
"joke": "Everything King Midas touches turnes to gold. Everything Chuck Norris touches turns up dead."
}
}
# Example: Request with parameters
curl https://api.icndb.com/jokes/random?limitTo=nerdy | python -m json.tool
# Response
{
"type": "success",
"value": {
"categories": [
"nerdy"
],
"id": 493,
"joke": "Chuck Norris can binary search unsorted data."
}
}
Authentication required
• Example: RESTCONF
# Example 3: Network Programmability with RESTCONF
curl -vk \
-u root:D_Vay\!_10\& \
-H 'accept: application/yang-data+json' \
https://ios-xe-mgmt.cisco.com:9443/restconf/data/ietf-interfaces:interfaces/interface=GigabitEthernet2
• -v verbose mode
• -k accept the self-signed certificate
• -u provides user:password for Basic authentication
• -H to set headers
# Request Header
> GET /restconf/data/ietf-interfaces:interfaces/interface=GigabitEthernet2 HTTP/1.1 # HTTP verb
> Host: ios-xe-mgmt.cisco.com:9443
> Authorization: Basic cm9vdDpEX1ZheSFfMTAm
> User-Agent: curl/7.52.1
> accept: application/yang-data+json
>
• Lines beginning with “>” indicate Request elments
# Response Header
< HTTP/1.1 200 OK # Response status
< Server: nginx
< Date: Tue, 30 Apr 2019 03:43:59 GMT
< Content-Type: application/yang-data+json # Content type
< Transfer-Encoding: chunked
< Connection: close
< Cache-Control: private, no-cache, must-revalidate, proxy-revalidate
< Pragma: no-cache
<
• Lines beginning with “<” indicate Response elments
# Response Data
{
"ietf-interfaces:interface": {
"name": "GigabitEthernet2",
"description": "Configured by RESTCONF",
"type": "iana-if-type:ethernetCsmacd",
"enabled": true,
"ietf-ip:ipv4": {
"address": [
{
"ip": "10.255.255.1",
"netmask": "255.255.255.0"
}
]
},
"ietf-ip:ipv6": {
}
}
}
Index