Pipes
• Use the output from one program as the input of the other program
• Syntax: prog1 | prog2
# List python script files in multiple directories
ls /bin /usr/bin | sort | uniq | grep -F ".py"
# or
ls /bin /usr/bin | sort -u | grep -F ".py"
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Redirection
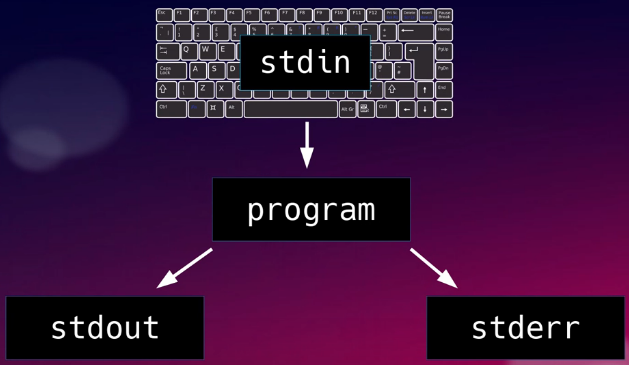
• Every process run by the Linux kernel has 3 channels
◇ The standard input (stdin)
▪ stdin is the keyboard by default. It has a file descriptor of 0
◇ The standard output (stdout)
▪ stdout is the shell by default. It has a file descriptor of 1
◇ The standard error (stderr)
▪ stderr output is the shell by default. It has a file descriptor of 2
• Redirection can be used with && (see Filtering)
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Output Redirection
• To redirect the output Use > (to overwrite) or use >> (to append)
# Redirect stdin to a file
echo "This is line 1" 1> somefile
# or
echo "This is line 1" > somefile # overwrite
echo "This is line 2" >> somefile # append
# Redirect stderr to a file
ls -l /dir_not_exist > bin.out
cat bin.out # nothing in file bin.out
ls -l /dir_not_exist 2> bin.out
# Redirect both stdin and stderr to a file
ls -l /home /dir_not_exist &> err.out
# Redirect stdin to /dev/null (discard)
ls -l /usr/bin &> /dev/null
# Useful when you run some lengthy job on a server and the output is not needed or you do some programming and you want to discard the output
# Concatenate files
cat file1.txt file2.txt > combine.txt # cat - handle input
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Input Redirection
• To redirect input , use <
# Send an email with a log file to the administrator
mail -s "This is the subject" to_username < message_log.txt
wc -l < testfile
# or
cat testfile | wc -l
• Without tee, if you want to capture an output to a file and stdout, you need to open two shells
# Shell #1
touch mylog.log
echo date >> mylog.log
date
cat mylog.log
# Shell #2
tail -f mylog.log
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
tee
• With tee, you can redirect the output to 2 places: stdout and a file
# Use tee to save intermediate results to a file
ls /bin /usr/bin | sort -u | tee sorted.txt | grep -F ".py"
# Watch & Log Command Output
date | tee mylog.log
date | tee -a mylog.log (append) # alternate
cat mylog.log
Index