Check Linux Version
uname -sr
uname -r
cat /etc/os-release
cat /etc/debian_version
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Basic Commands
cd - # go back to previous working directory
echo > file.txt # same as touch file.txt
file filename.jpg # show info on a file
mkdir -p parent/dir0 # create nested directory
touch file # change the modification time of existing file
cp -a file file1 # archive: preserve attribute like time of file
cp -i file file1 # interactive: prompt for overwrite
cp -uv file*.csv dir1/ # copy only modified files use with -v for verbose
# alternative: use rsync command
rm -rf / # remove everyting in your system !
rm -i file*.txt # -i - interactive - suggest to use with rm
rmdir dir2 # can't remove, directory not empty
rm -ivr dir2 # used to remove non-empty directory
rm -rf dir1 # force remove (-r == -R)
ls
# -S # sort (largest first)
# -h # size in human readable form
# -r # reverse
ls [Ff]* # list files start with f or F
ls [!Ff]* # list files not start with f or F
ls FILE??.txt # list files named FILE with 2 characters
history # show command history
!nan # execute last command starts with "nan"
!13 # execute line number 13
systemctl status
systemctl list-unit-files
systemctl enable servicename
systemctl start servicename
systemctl stop servicename
systemctl restart servicename # systemd only
ifconfig
ip a
curl ifconfig.me # Get external ip address
tail -f /var/log/demesg # follow log file update in real time
whoami
id # user id and groups
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Finding/ Searching FIles and Others
• Syntax: find path -iname exact_filename or filename*
find ~/dir -iname media* # iname - case insensitive
find ~/Downloads/ -mtime -7 # find files in the last 7 days
find ~/Downloads/ -mtime +7 # find files before the last 7 days
find ~/Downloads/ -mmin -7 # find files in the last 7 minutes
# find files in the last 30 days and above 10MB in size
find ~/Downloads/ -mtime -30 -size +10M
# find executables, man pages, sources
whereis gimp
which gimp
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
About $PATH in Bash
• To avoid injection of evil shell script, always put the full (absolute) path in script files
echo $PATH
$PATH=$PATH:/some/dir # Prepend to $PATH
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Environmental Variables
echo $USER # returns your user name
printenv | less # get the full list
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Hard & Soft Links
• Hard link
◇ valid within the same file system
◇ can't link to a directory
• Soft / Symbolic link
◇ can span file systems
◇ can link to a folder
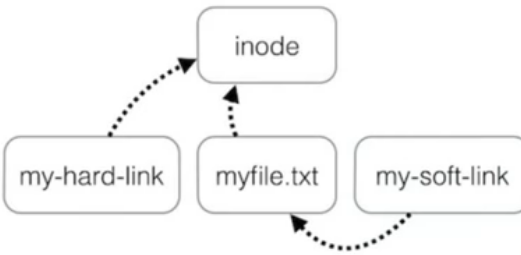
cd Public
echo hard > file1
echo soft > file2
ln file1 hardLink # create a hard link to file1
ln -s file2 softLink # create a soft link to file 2
cat hardLink # same as cat file1
cat softLink # same as cat file2
mv file1 file1.1
mv file2 file2.1
cat file2 # link lost, no content
cat hardLink # Still exist content (link to inode)
rm file1.1
cat hardLink # still exist
rm hardLink # remove hardLink, inode is gone
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Alias
• Create your own command
• To make alias peristent:
◇ Add the above line to nano ~/.bashrc or /etc/bash.bashrc
◇ Logout and login
alias # List existing alias
alias ll='ls -l' # Create new alias
ll
unalias ll # Unset/ remove alias
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Echo
echo $SHELL # shell currently using
echo Some text > file.txt # save some text in a file
echo $((2+6)) # returns 8
echo There are 3 spaces here # There are 3 spaces here
echo "There are 3 spaces here" # There are 3 spaces here
echo two plus one = $((2+1)) # two plus one = 3
echo "two plus one = $((2+1))" # two plus one = 3
echo 'two plus one = $((2+1))' # two plus one = $((2+1))
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Escape Character \
# Escape character
echo this cost $10.00 # this cost 0.00
echo this cost \$10.00 # this cost $10.00
echo you & me # error
echo you \& me # you & me
man echo | grep '\\' # include lines with \
echo -e "line 1\nline2" # use -e when you use \n
# line1
# line2
echo line 1$'\n'line 2 # alternate
# line1
# line2
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Bracket expansion
echo {A..Z} # print alphbets A to Z
echo number_{1,3,4} # number_1 number_3 number_4
echo number_{1..5}
touch number_{1,3,4}
mkdir number_{1,3,4}
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Reverse Mouse Button
xmodmap -e "pointer = 3 2 1"
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Stream Editor (SED) Find & Replace
# Stdout
ysed 's/search-str/replace-str/g' filename
# Write to filename
sudo sed -i 's/search-str/replace-str/g' filename
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Why Doesn't ‘sudo cd /var/named’ work?
• Command cd is not a program but an built-in command
• Command sudo only applies to programs
• Workaround:
sudo -i # elevate to root
cd /var/named
exit
Set Time Zone
sudo dpkg-reconfigure tzdata # reboot
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Example Usages
• Copy specific files from many directories
# From many directories to one directories
cp dir/*/*.png Pictures/Thumbnails/
• Convert many MOV videos to mp4
for i in *.MOV; do ffmpeg -i $i -vcodec h264 -acodec aac -strict -2 $i.mp4; done
• Resize many images at once
for i in *.jpg; do convert -resize 50% $i re_$i; done
• Rename many files
# Change extension of txt to csv for all txt files
rename txt csv *.txt
• Check bitrate
exiftool -AudioBitrate unknown.mp3 # single file
exiftool -AudioBitrate *.mp3 # all files
• Work with really large files
# Open a gz file with > 100GB
zcat filename.gz | less
• Replace a string in many files
wc -l file.csv # number of lines
head file.csv # top 10 lines
for i in *.csv; do sed -i 's/search_str/replace_str/g' $i; done
• Impress your friends
sudo apt install hoolywood wallstreet # available in unstable & testing depo
sudo apt install cmatrix
Index